Google reCAPTCHA v3 PHP Ajax Example (GitHub Link)
If you have a website, you need to protect your users from bots. Bots are trying leaked passwords, posting spam & scraping your content. The reCAPTCHA “I’m not a robot” checkbox changed the way to protect your website. Google reCAPTCHA v3 does away with the need for interactive tests and gives you a score to let you know when you have risky traffic. It never interrupts users so you are in control of when to run risk analysis and what do to with the results. For instance, requiring email verification for risky logins, sending a spammy post to moderation, or filtering fake friend requests. With Google reCAPTCHA v3, you can keep your site safe without user friction and get more control to stop attacks in your own way. To know more about Google reCAPTCHA v3 please visit the google official site here. Its a totally free service of Google.
In this tutorial, I am going to show you how to integrate Google reCAPTCHA v3 on your website to protect your users from bots. There are three-part of this tutorial.
- Firstly, Register your website to get SITE KEY & SECRET KEY
- Secondly, Client-side Integration
- Finally, Server-side Integration
Register your website
At first, you have to register your website on Google reCAPTCHA admin console to collect SITE KEY & SECRET KEY. To do that please click here.
You have to log in to your Google account to access the reCAPTCHA admin console. After then fill-up the form properly. Specify a label that will make it easy for you to identify the site in the future. Select reCAPTCHA type v3 & your hostname (website address: example.com). If you want to develop this reCAPTCHA from your local development area then you can add just “localhost” for instance. Also, you can add multiple hostnames where the reCAPTCHA will verify each request. Make sure that no need to add extra path or protocol or any port like https://example.com/website/index.php. Just add exapple.com.
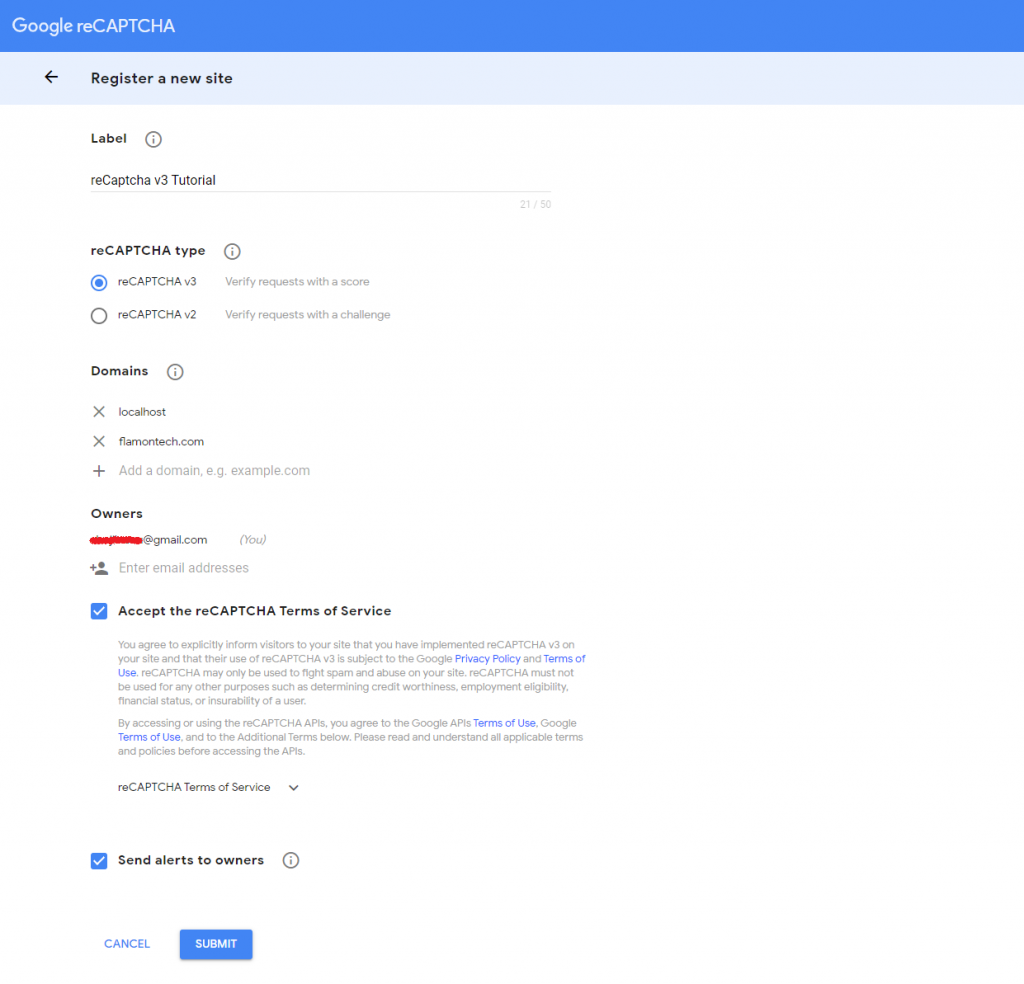
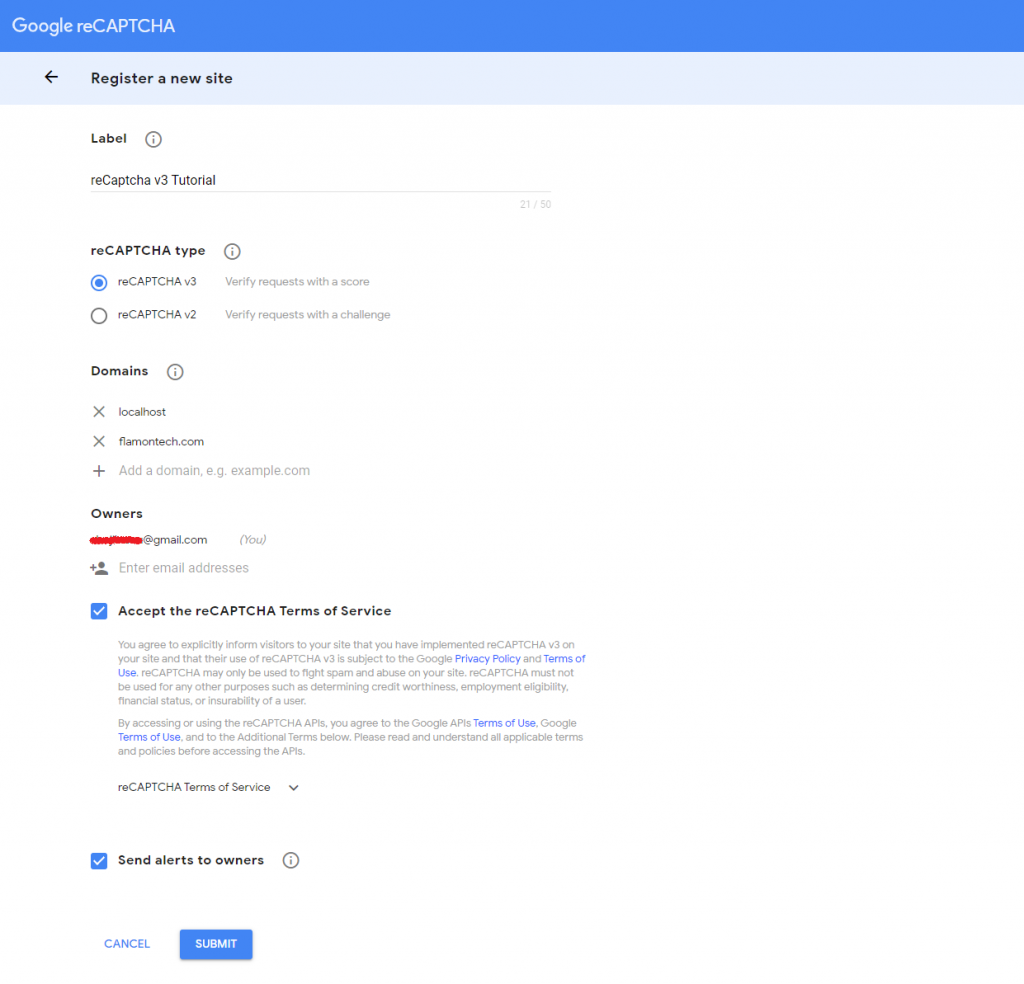
Once submitted your website’s information, Google will provide you following two pieces of information. Those two pieces of information are responsible to connect your website with Google reCAPTCHA v3. Don’t share that information publicly.
- SITE KEY
- SECRET KEY
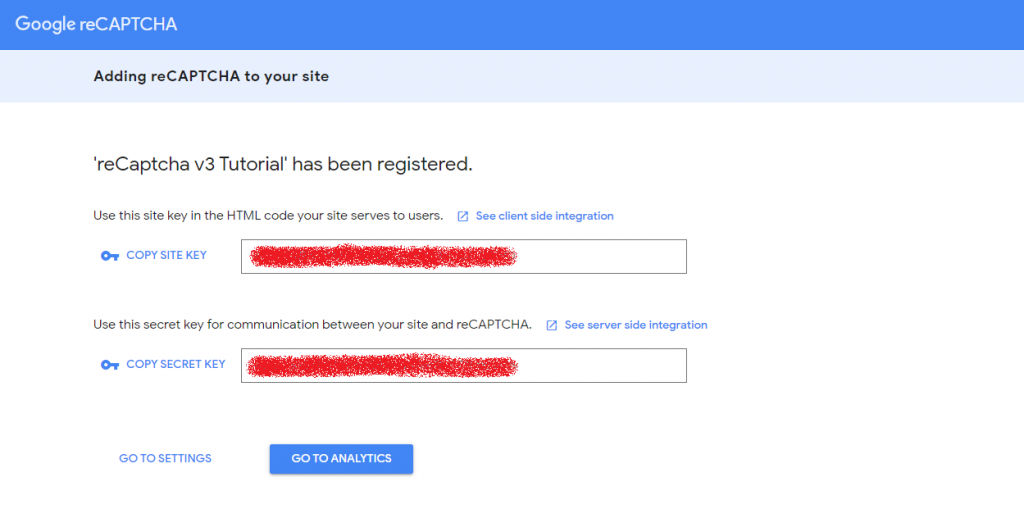
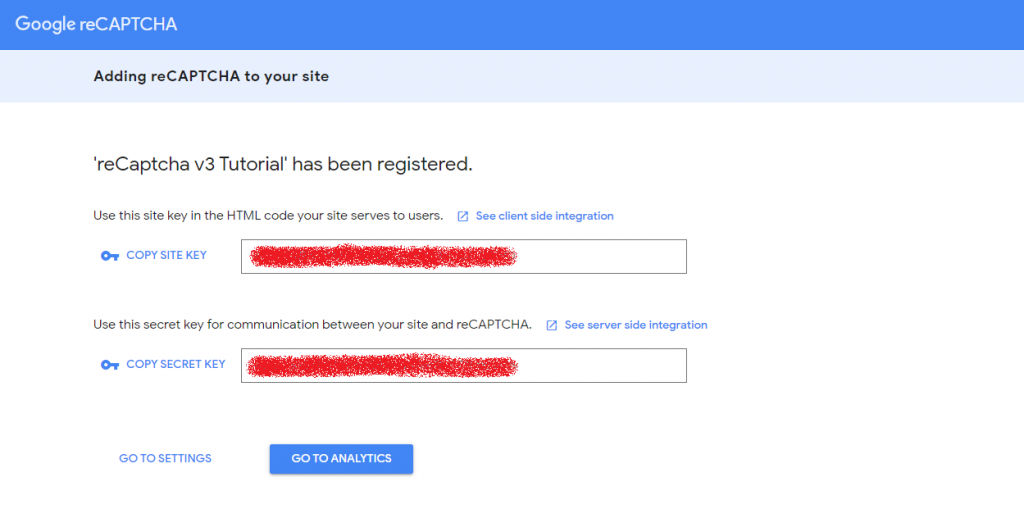
Client-side Integration
Firstly, you have to load Javascript API with your SITE KEY. To do this you need to add a render parameter to the reCAPTCHA script load in your website head section.
1 2 3 |
<script src="https://www.google.com/recaptcha/api.js?render=reCAPTCHA_site_key"></script> |
Secondly, create a registration form. You can add some other CSS or javascript library whatever you need with your template. For example, I will just add the bootstrap CSS library to view them from more beautiful. In the script section, you have to add ajax function on your form submission. You need to call grecaptcha.execute on each action you wish to protect. Here I will protect the User Registration from the action. That’s why I will add this execute method of grecaptcha object on ajax submit. When call the execute method a token will receive and bind it with from and Finally, send that token immediately to your backend with the request to verify. That verification part is called Server-side Integration.
Registration Html Form (index.php)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
<!DOCTYPE html> <html> <head> <title>Google Recaptcha v3</title> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> <script src="https://www.google.com/recaptcha/api.js?render=reCAPTCHA_site_key"></script> <link href="style.css" rel="stylesheet"> <script src="javascript.js"></script> </head> <body> <main class="py-4"> <div class="container"> <div class="card"> <div class="card-header"> Registration Here </div> <div class="card-body"> <!-- Image loader --> <div id="loader"> <img src="loder.gif"> </div> <!-- Image loader --> <div class="row justify-content-center"> <div class="col-6"> <!-- Response Notification --> <div id="response_notification"></div> <!-- Response Notification --> </div> </div> <div class="row justify-content-center"> <div class="col-6"> <form method="post" id="myLoginForm"> <div class="form-group"> <label>Full Name</label> <input type="text" class="form-control" placeholder="Full Name" name="fullname" required> </div> <div class="form-group"> <label>User Name</label> <input type="text" class="form-control" placeholder="User Name" name="username" required> </div> <div class="form-group"> <label>Password</label> <input type="password" class="form-control" placeholder="Password" name="password" required> </div> <input type="hidden" name="g-recaptcha-response" value="" id="g-recaptcha-response"> <input type="submit" class="btn btn-black" value="Submit"> </form> </div> </div> </div> </div> </main> </div> </body> </html> |
CSS (style.css)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
#myLoginForm { padding: 55px 0px; } #loader { height: 256px; width: 256px; margin: 0 auto; position: fixed; z-index: 9999; top: 150px; right: 0; left: 0; display: none; } .btn-black{ background-color: #000 !important; color: #fff; } .grecaptcha-badge { bottom: 100px !important; } |
The Registration form looks like this.
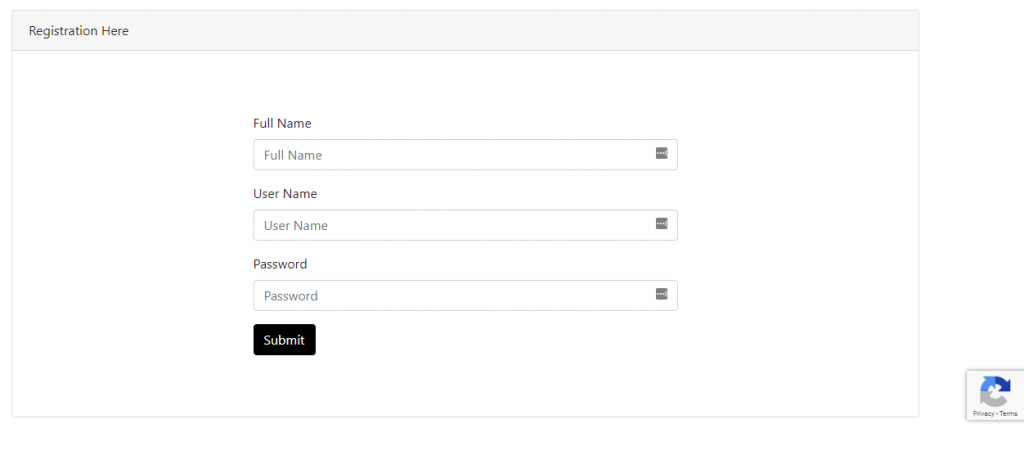
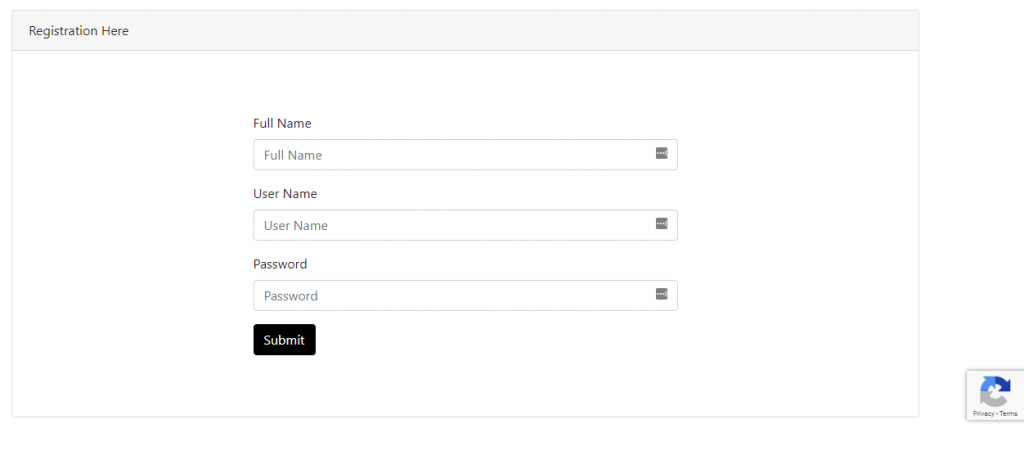
Here is the Javascript file where you have to generate token and bind it with from for sending token to server end.
Javascript (javascript.js)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
$( document ).ready(function() { var request; $('#myLoginForm').submit(function(event){ // Prevent default posting of form - put here to work in case of errors event.preventDefault(); // Abort any pending request if (request) { request.abort(); } var $form = $(this); grecaptcha.ready(function () { grecaptcha.execute('reCAPTCHA_site_key', {action: 'submit'}).then(function (token) { $('#g-recaptcha-response').val(token); var serializeDataArray = $form.serializeArray(); // console.log(serializeDataArray); $.ajax({ type: 'POST', url: 'post.php', data: serializeDataArray, dataType: 'json', beforeSend: function () { $("#feedback").css("opacity", 0.3); $('#loader').show(); }, success: function (data) { console.log(data); if (data['status']) { $('#response_notification').html('<div class="alert alert-success" style="display:none;"><a class="close" data-dismiss="alert" href="#">×</a>' + data['message'] + '</div>'); } else { $('#response_notification').html('<div class="alert alert-danger" style="display:none;"><a class="close" data-dismiss="alert" href="#">×</a>' + data['message'] + '</div>'); } }, complete: function () { $('#loader').hide(); $("#feedback").css("opacity", 1); $('.alert').fadeIn('slow'); } }); }); }); }); }); |
Server-side Integration (post.php)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
<?php define('RECAPTCHA_V3_SECRET_KEY','reCAPTCHA_secret_key'); if($_SERVER['REQUEST_METHOD'] == 'POST'){ $res = verifyReCaptchaV3(); if($res['success']){ $fullname = $_POST['fullname']; $username = $_POST['username']; $password = $_POST['password']; $g_recaptcha_response = $_POST['g-recaptcha-response']; // REGISTER YOUR USER IN YOUR DATABASE WITH VALIDATION // CODE HERE // REGISTER YOUR USER IN YOUR DATABASE WITH VALIDATION // RETURN JSON RESPONSE $json = [ 'status' => true, 'message' => 'Registration Successfull. Hello, Mr. ' . $fullname, 'reCaptchaResponse' => $res, ]; }else{ $json['status'] = false; $json['message'] = 'There is some error. Please try again.'; } echo json_encode($json); } function verifyReCaptchaV3(){ $site_verify_url = "https://www.google.com/recaptcha/api/siteverify"; $data = [ 'secret' => constant('RECAPTCHA_V3_SECRET_KEY'), 'response' => $_POST['g-recaptcha-response'], // 'remoteip' => $_SERVER['REMOTE_ADDR'] ]; $options = array( 'http' => array( 'header' => "Content-type: application/x-www-form-urlencoded\r\n", 'method' => 'POST', 'content' => http_build_query($data) ) ); $context = stream_context_create($options); $response = file_get_contents($site_verify_url, false, $context); $res = json_decode($response, true); return $res; } ?> |
After submitting the form, the server-side PHP code will verify that token with Google reCAPTCHA v3 verify the link and give a score & status. Depending on that status or score you can set your logic to save data into the database & complete the user registration process. That score & status detect the user’s behavior that the user who put the information in the registration form is human or bot.
In conclusion, Here is the final complete registration process.
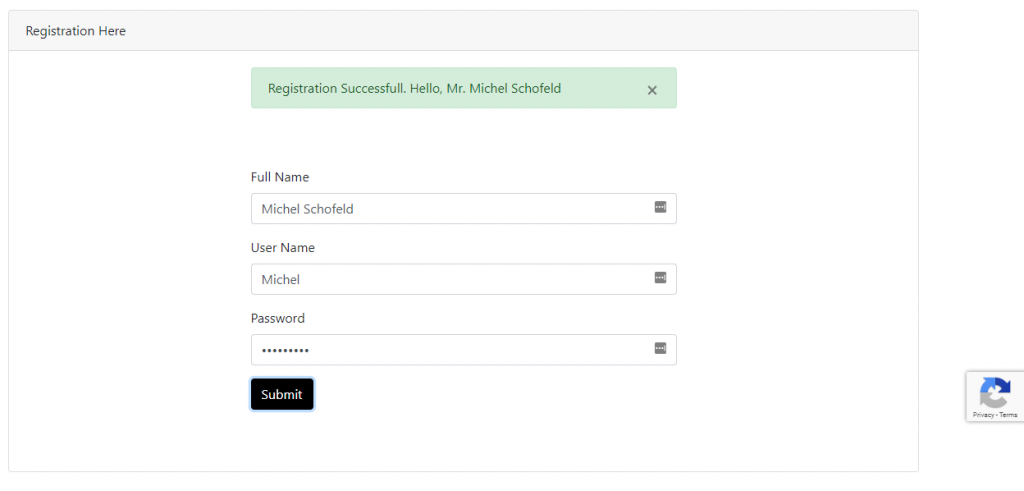
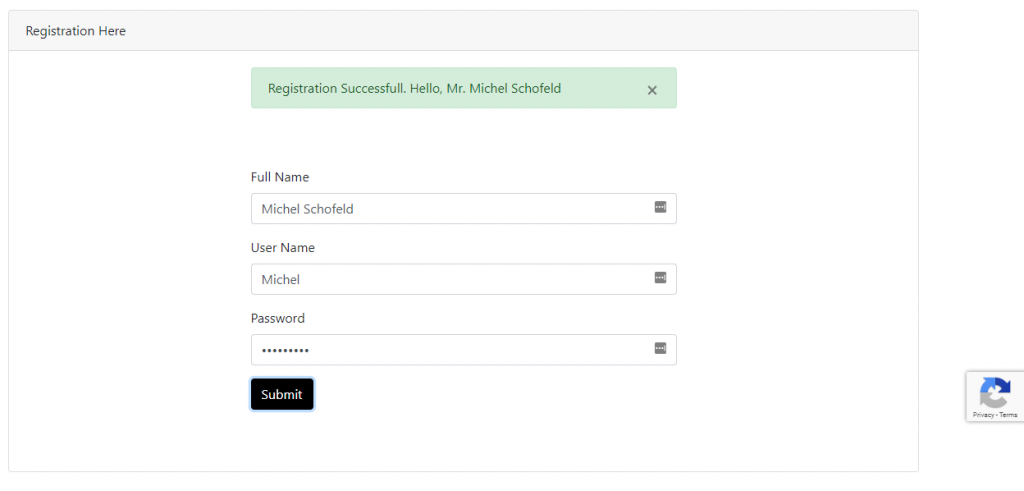
You can check the Demo link here for the sample. Moreover, clone the GitHub link here for getting the complete source.
This tutorial is really good with an explanation. You can also check another article to add Google reCAPTCHA V3 in PHP contact form with a live demo and can download complete code.
Thanks
Excellent. you can also check another article on how to integrate google recaptcha v3 in php with live good results.