Unzip your zip archive file using raw php
Sometimes you need to extract or unzip a zip file in your application. Such as different types of plugins or any other thing. So in this article, I am going to tell you how to unzip a zip file using raw PHP. You don’t need to use any third-party plugins.
Server Requirement :
- PHP Version : (PHP 5 >= 5.2.0, PHP 7, PECL zip >= 1.1.0)
- Must have to enabled ZipArchive extension in php, otherwise you will get some Fatal Error : Class ‘ZipArchive’ not found in /home/Username/public_html/….
Php has a ZipArchive Class that actually helps to create or extract a zip file. I am going to use three methods of this class to unzip a zip file. More information please check here
First one is open() method, Second one is extractTo() method and third or last one is close() method.
Method open(): It’s actually open the zip archive file and return a boolean true value if this method opens this zip file correctly. Otherwise, this method will return an error code.
Method extractTo() : This method have only one parameter & that is destination. After getting boolean value true from open() method then have to call extractTo() method. This method will extract or unzip this file to that given destination (path or directory where you have to unzip).
Method close() : Close method actually complete those unzip process.
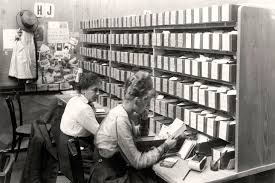
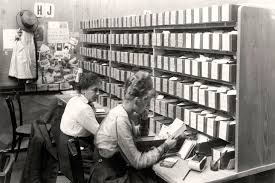
Unzip archive file
Here is the sample code to extract or unzip a particular zip archive file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<?php // DISPLAY ERROR IN DEVELOPMENT MODE // FOR PRODUCTION MODE ITS NOT RECOMMENDED ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL); // CURRENT DIRECTORY OR YOUR PROJECT DIRECTORY define('_PATH', dirname(__FILE__)); // EXTRACT PATH WHERE YOU ZIP FILE WILL BE UNZIPPED $zip_extract_directory = _PATH.'/extract_items'; // ZIP FILE NAME $zip_file_name = 'sitemap.zip'; $zip = new ZipArchive; $response = $zip->open($zip_file_name); if ($response === TRUE) { echo 'SUCCESSFULLY UNZIPPED'; $zip->extractTo($zip_extract_directory.'/sitemap'); $zip->close(); } else { echo 'UNABLE TO EXTRACT YOUR ZIP FILE , ERROR CODE :' . $response; } ?> |
Conclusion :
Using this PHP script you can extract your zip file to any location. This script is for sample understanding for extracting zip files. For your custom requirement, you have to add some extra code like if you want to validate zip file then you have to add some extra code to validate zip file that the file was .zip extension or not or other things. Also, you can make a package using this class in any framework like laravel or any other custom framework or CMS.
If you have some more query then please feel free to reply to me in the comment box. I will always beside you.
Enjoy your coding……….
Recent Comments